About the project
Welcome to my Project Portfolio Page! My team and I were tasked to enhance an open source, basic command line interface (CLI) addressbook for our Software engineering project. Given the constraints that the final product had to use CLI as the primary mode of input and be functional offline, we decided to morph it into a project management application called Pocket Project.
This upgraded application enables project managers to keep track of everything about ongoing and completed projects, as well as the data of employees under the manager, all in one place.
My role was to design and write the code for the addto projecttask
, update projecttask
and removefrom projecttask
features. The following sections will explain in more detail these features,
as well as my documentation contributions in the user and developer guides during this project.
Before we continue, do take note of the following symbols and formatting used in this document:
Symbol | Meaning |
---|---|
The note pad icon indicates any information which you might find handy. |
|
The light bulb icon indicates any shortcuts that you can use while using application. |
|
The exclamation mark icon indicates any warnings that you might want to take note of when using Pocket Project. |
|
|
A grey highlight (called a mark-up) indicates that this is a command you can use in Pocket Project. In the documentation sections, this might refer to code-related implementations in the application. |
|
A grey highlight with light blue text indicates this is an abbreviated command shortened for the sake of brevity. |
Summary of contributions
This section shows you a summary of my coding, documentation, and other helpful contributions to the team project.
Major feature added: Project tasks which helps users to track a project’s progress.
Milestones give a broad overview of what is needed to complete a project. Project tasks in milestones help keep track of what needs to be done to reach the next milestone. |
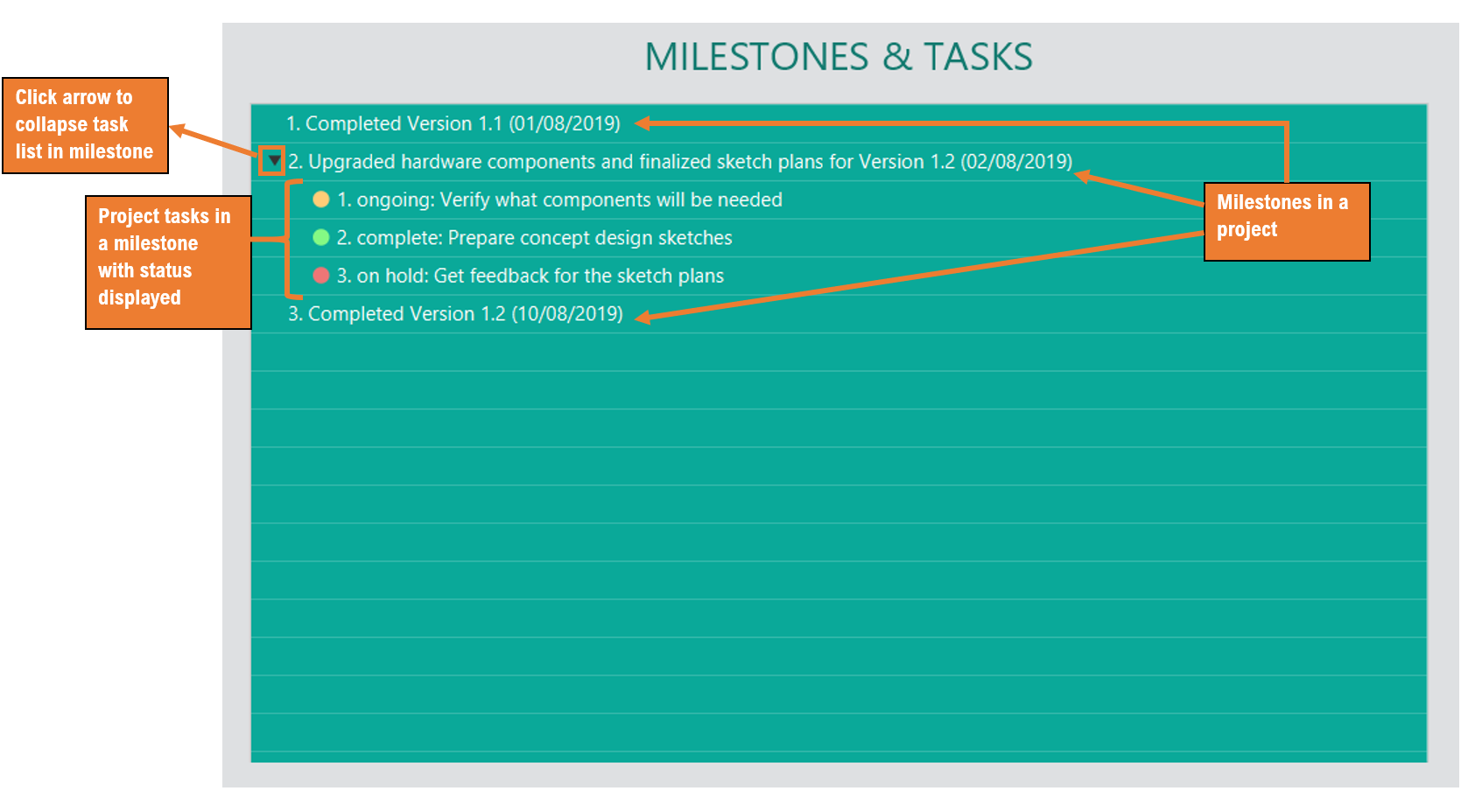
-
What it does: The
addto projecttask
command adds a task to a milestone in a project. Theupdate projecttask
command allows the manager to set the status of the task to ongoing, on hold or complete. Theremovefrom projecttask
command removes the specified task completely from the milestone. -
Justification: This feature improves the product significantly because it greatly enhances the level of organization and control which the manager has while planning out a schedule for projects.
-
Highlights: The implementation of this feature was slightly unique and required an approach different from how other features were implemented as a project task is a component of milestones which is in a project. Most other features in Pocket Project directly interact with either employees or projects, unlike that of project tasks which interact only with milestones. It required me to make changes to the behaviour of the existing Milestone class and how it was stored in a project in order to get it to work as desired.
-
Credits: The code for
addto
command parser which I extended for the project task feature and the original milestone code which I enhanced was done by my teammate Jothinandan Pillay. The code forupdate
command parser was done by my teammate Jeff Gan. The code forremovefrom
command parser was done by my teammate Lin Dehui. -
Code contributed for project task feature
Other contributions:
-
Project management:
-
Managed releases
v1.2
-v1.3.1
(3 releases) on GitHub -
Set up kanban with automation on Github
-
-
Minor code changes:
-
Team contributions:
-
Community contributions:
Contributions to the User Guide
You can view this section to see my contributions to the User Guide documentation for addto projecttask
, removefrom projecttask
and update projecttask
command.
Add a project task to a project’s milestone: addto projecttask
Building further on the functionality of milestones, you can also add project tasks to existing milestones in Pocket Project. This allows you to track and plan out in more detail what needs to be done between each milestone.
Format: addto PROJECT_NAME projecttask n/PROJECT_TASK_DESCRIPTION m/MILESTONE_INDEX
The |
Examples:
-
addto Apollo projecttask n/Create feature XYZ m/1
A project task with the given description will be added to the list of tasks under the milestone at index 1 in project "Apollo". -
addto Orbital projecttask m/2 n/Prepare concept design sketches
A project task with the given description will be added to the list of tasks under the milestone at index 2 in project "Orbital".
There is no specific order for either the project task name or the milestone index when entering the command so either way is acceptable. |
You can refer to the screenshot below to have a clearer picture of how it works and looks like.
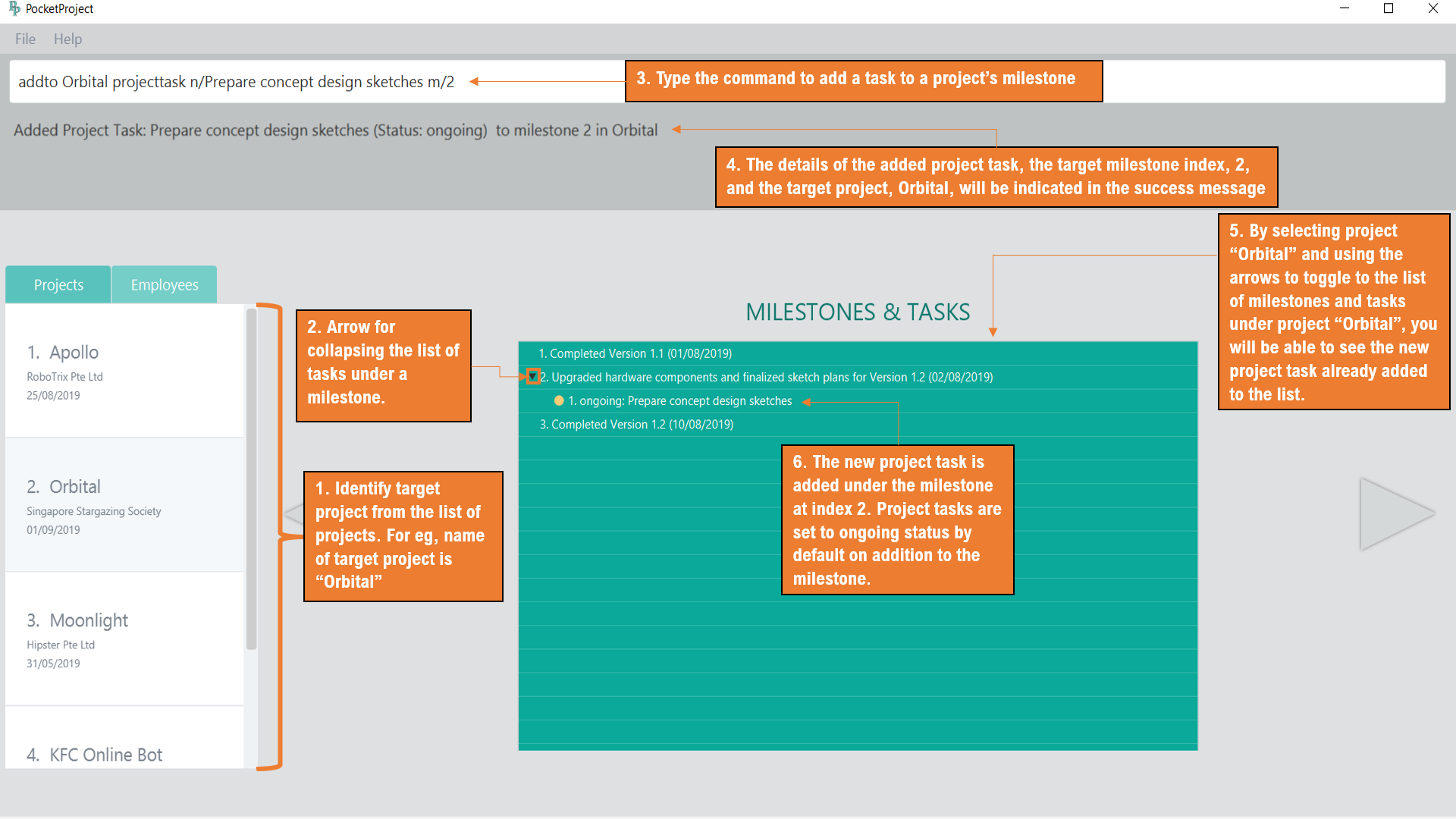
Clicking on the arrow beside a milestone containing existing tasks allows you to hide the list of tasks under the milestone. |
Remove a project task from a project’s milestone: removefrom PROJECT_NAME projecttask
You can remove existing project tasks from a milestone if there is a change in the planned project’s schedule.
Format: removefrom PROJECT_NAME projecttask MILESTONE_INDEX PROJECT_TASK_INDEX
The |
Examples:
-
removefrom Apollo projecttask 1 1
The 1st project task in the task list under the 1st milestone of the displayed milestone list in project "Apollo" will be removed. -
removefrom Orbital projecttask 2 1
The 1st project task in the task list under the 2nd milestone of the displayed milestone list in project "Orbital" will be removed.
You can refer to the figure below to have a clearer picture of how it looks like when the command removefrom Orbital projecttask 2 1
is called.
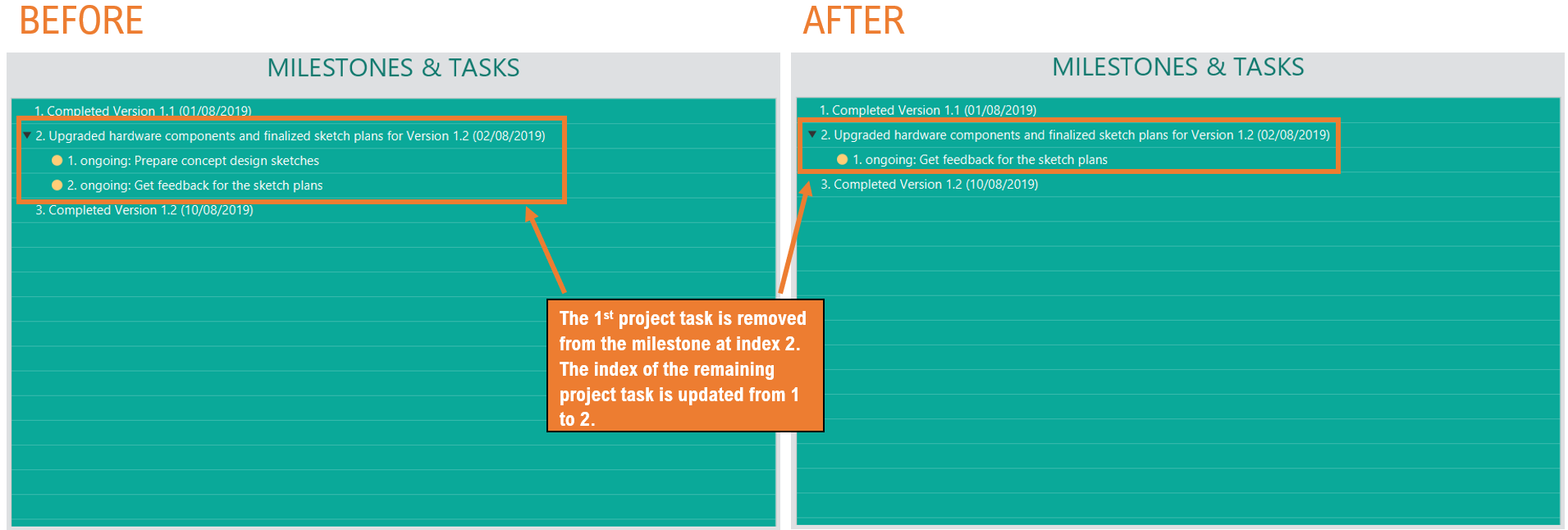
The arrow beside the milestone description for collapsing the task list will disappear if all tasks for that milestone are removed. |
Update the status of a project task: update projecttask
You can update the status of a project task to indicate its progress.
Format: update PROJECT_NAME projecttask MILESTONE_INDEX PROJECT_TASK_INDEX STATUS
The |
Examples:
-
update Apollo projecttask 1 1 ongoing
Updates the status of the first project task in the first milestone of project 'Apollo' to "ongoing". -
update Orbital projecttask 2 1 complete
Updates the status of the first project task in the second milestone of project 'Orbital' to "complete".
You may refer to the diagram below to get a clearer picture of how updating a project task’s status looks like when the command update Orbital projecttask 2 1 complete
is called.
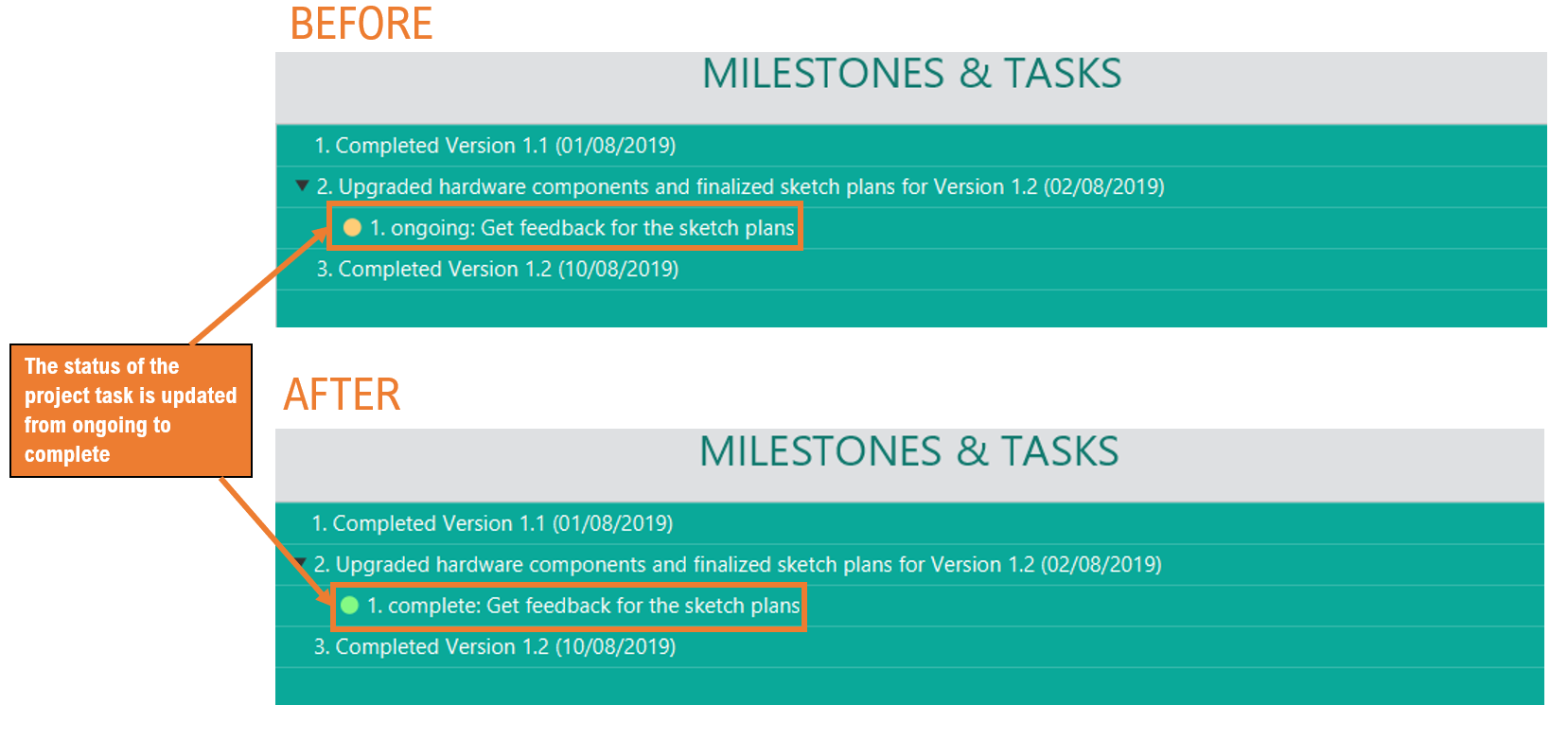
Contributions to the Developer Guide
You can view this section to see my contributions to the Developer Guide documentation which consists of the implementation of the project task feature and also several sections on manual testing Pocket Project (which unfortunately I am out of space to include in here, so check out this link if you’re interested! [Manual testing, sections G3-G6, G12-G13).
Project Task feature
Current Implementation
This project task feature enhances the usage of the milestone feature in Pocket Project for keeping track of a project’s schedule by providing you with a list of tasks which needs to be done between milestones.
The project task feature is mainly facilitated
by the model component, and in particular, the Milestone
class which stores the relevant project tasks in a UniqueProjectTaskList
.
This feature supports three main commands:
-
addto [project name] projecttask n/PROJECT_TASK_DESCRIPTION m/MILESTONE_INDEX
— Adds the project task with the given[PROJECT_TASK_DESCRIPTION]
to the milestone specified by[milestone index]
in the project named[project name]
. -
removefrom [project name] projecttask [milestone index] [project task index]
— Removes the project task at the specified[project task index]
from the milestone specified by[milestone index]
in the project with name[proejct name]
. -
update [project name] projecttask [milestone index] [project task index] [status]
— Updates the status of the project task at the specified[project task index]
in the milestone specified by[milestone index]
in the project with name[project name]
.
Project tasks contain statuses to help you oversee its progress, whether its "Ongoing", "On hold" or "Complete".
Implementation of project task commands
The project task commands are supported by the methods in the Model
interface: Model#addProjectTaskTo(Project, Milestone, ProjectTask)
, Model#removeProjectTaskFrom(Project, Milestone, ProjectTask)
and Model#updateProjectTask(Project, Milestone, ProjectTask, Status)
.
These three methods take in Project
, Milestone
and ProjectTask
as common arguments. Hence, the correct project/milestone/project task which the commands act on are first located through
AddProjectTaskToCommand#excute()
, RemoveProjectTaskFromCommand#execute()
or UpdateProjectTaskCommand#execute()
before the methods of Model
acting on the milestone/project task are called.
Update status of project task
Given below is an example usage scenario and a showcase of how a project task status is updated in a project’s milestone at each step.
-
update [project name] projecttask [milestone index] [project task index] [status]
— Updates the status of the project task at the specified[project task index]
in the milestone specified by[milestone index]
in the project with name[project name]
.
The sequence diagram for the execution of updating a project task status is as follows:
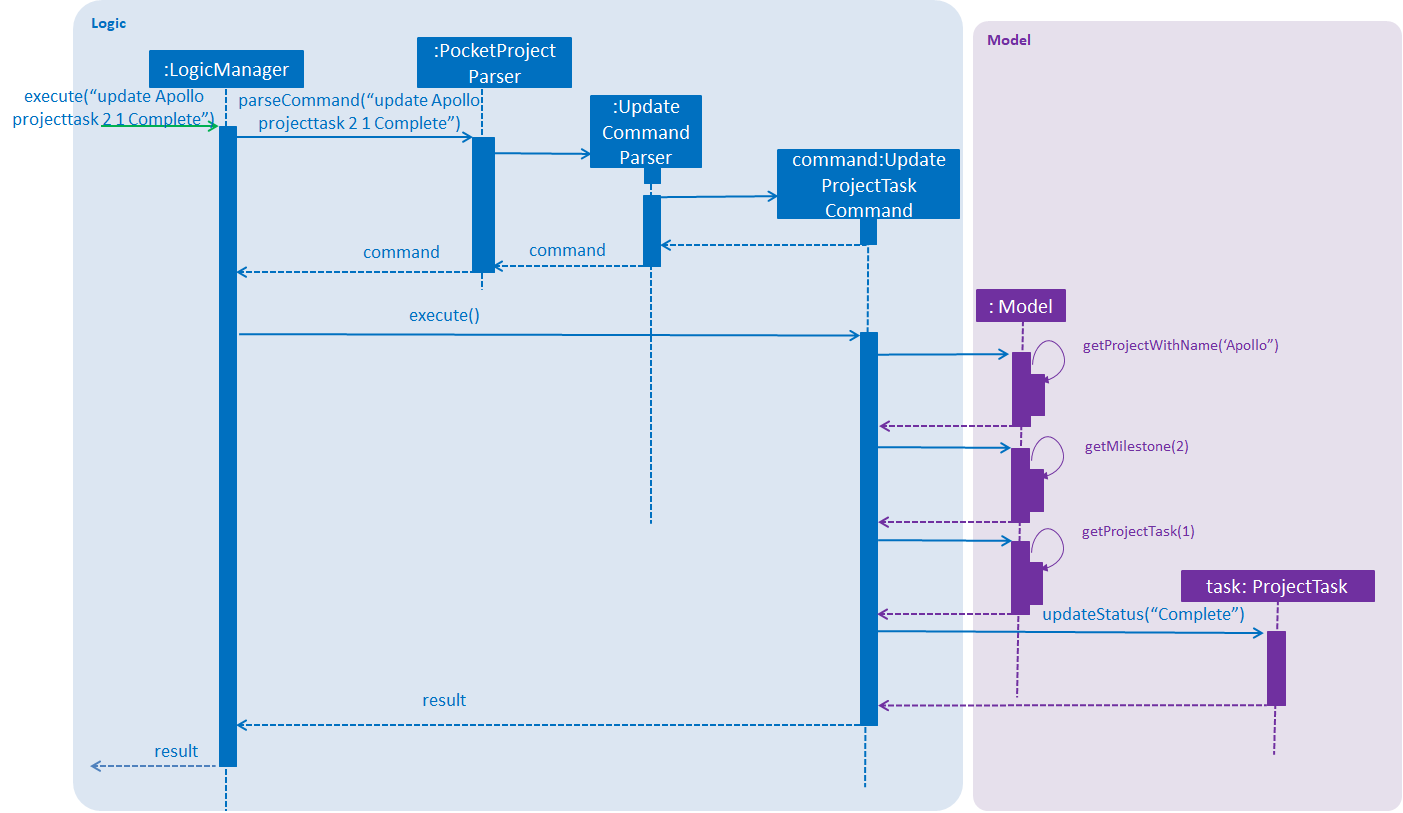
update Apollo projecttask 2 1 Complete
Step 1. The user enters the command update Apollo projecttask 2 1 Complete
.
The LogicManager
then passes the entered string to the PocketProjectParser
. The PocketProjectParser
parses the string received and identifies the command as falling under the class of UpdateCommand
and passes the rest of the string to the UpdateCommandParser
to identify which type of UpdateCommand
is being executed and what are the arguments.
Step 2. The UpdateCommandParser
then identifies the "projecttask" keyword and creates an UpdateProjectTaskCommand
and passes it to the LogicManager
to be executed.
Step 3. On execution of the command, the validity of the arguments are checked and then call the methods of the Model
component to locate and obtain the necessary objects.
Step 4. The Model
component then searches for the project with name Apollo
. After which, it searches for the milestone at index 2 of the project’s milestone list. Then, it retrieves the specified project task at index 1 in the milestone’s project task list.
In each of these 3 searches for the specified object, after the object is found, it is returned to the UpdateProjectTaskCommand
object and used for the next search.
Step 5. The update status method of the retrieved task is then called with "Complete" as an argument. This updates the project task status to "Complete" and execution of the UpdateProjectTaskCommand
ends.
The |
Design Considerations
In this section, I will talk about certain alternatives that could have been taken when designing this feature and why I did or did not use them.
Aspect: How should project task objects be stored within a project
Alternative 1: Store project tasks in a UniqueProjectTaskList
which is in turn stored under a specified milestone. (Current implementation)
-
Pros: Only milestone objects are in direct contact with the project tasks through the
UniqueProjectTaskList
container class and checks to ensure project tasks work as they should only need to be done within the milestone instead of in the entire project itself. -
Cons: Somewhat harder to implement as it requires finding the correct milestone in a project first before anything can be done for the project task specified in the user’s command.
Alternative 2: Store project tasks directly in a project and keep a reference to the milestone which it should be under.
-
Pros: Easy to implement and will have similar behaviour to existing implementations of other objects stored directly in a project.
-
Cons: It can be confusing in the backend as the user can have tasks under different milestones with the same project task descriptions. This also goes against the Object-Oriented Programming(OOP) Guideline, Law of Demeter as project tasks only need to be in direct contact with milestones but not necessarily with projects themselves.
Based on the above pros and cons for each alternative, I decided on Alternative 1 for 2 reasons:
-
As it was in line with the OOP guidelines and principle.
-
As it allowed me to implement a more robust and efficient checking system for project tasks which was constrained within a milestone itself.
Future planned enhancements
The following features are scheduled to be implemented in a future version of Pocket Project:
-
Allow the assigning of project tasks to employees in a project which gives you a better overview of who is in charge of doing what task during the project’s duration.
-
Include the completion date of a project task when it is completed, allowing you to backtrack from the completion date of the project task to see what was done for the project task’s completion.