About PocketProject
My team and I were given the task to enhance or morph a basic command line interface Addressbook for our software engineering Project. Our team decided to morph the AddressBook into a project management tool named Pocket Project. Pocket Project is designed to suit the needs of software engineering managers by providing them a one-stop platform to store all the information that are essential for software engineering project planning in order to make planning projects easier and more convenient for them.
My team was inspired by our own struggle of having to manage multiple projects across different modules and decided to make PocketProject in hopes of having better project management when we start venturing into the working world as a software engineer.
My Role
My primary role in this project was to implement find
command which is essential in allowing users to navigate through the application and edit
command which is necessary as users need to change various information to ensure that things are always up-to-date.
Below are explanation of the symbols I used in this document.
Symbol | Meaning |
---|---|
The note pad icon indicates any useful tips or things to take note. |
|
The light bulb icon indicates any shortcuts that you can use while using the Pocket Project application. |
|
The exclamation mark icon indicates any warnings that you should take note of. |
|
|
The grey markup indicate that the term is either a command or the name of an object class. |
The following sections includes implementation done in details and also other contributions and relevant sections I have recorded in the user guide and developer guide.
Summary of contributions
This section shows a summary of contributions I have made for the project.
-
Major enhancement: I added the ability to search for projects and employees in the application.
-
What it does: The
find
command allows the user to search for projects and employees in the application by using different types of keywords such asname
,skill
, and more. -
Justification: Since the application contain so much information, it is difficult for the user to look through the whole list of employees or projects just to look for a certain project/employee. Hence this find command provide a convenient and fast way for the user to extract out the information needed.
-
Highlights: This command can be used together with other commands such as
edit
to filter out the employees or project before proceeding on to edit them. The command also support extension as new methods of finding can be added by using different command keywords.
-
-
Minor enhancement: I also added the ability to update the information of the various components inside the project.
-
What it does: The
edit
command allow users to edit the project information such as name, client, description and deadline as well as -
Justification: As the project progresses, there are bound to be changes made to the project information hence it is essential to have an
edit
command to make sure that all the information are up to date. -
Highlights: The parser for this command was difficult to implement because of the large number of argument inputs involved.
-
-
Other contributions:
-
Project management:
-
I managed releases version v1.1 and v1.2
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Tools:
-
Set up reposense
-
-
Contributions to the User Guide
This section includes User Guide documentation I have written for the features that I have implemented. Due to limited space I have extracted out the commands which are more distinct from the original AddressBook application.
Find employees with skills: find skill
You can look for employees by using their skills as keyword. This will make things easier for you when you need to find employees to assign to projects which require a certain skill.
Format: find skill KEYWORD [MORE_KEYWORDS]
Examples:
-
find skill java
Returns a list of employees who has java skill. -
find skill java C
Returns a list of employees who have either java or C or both.
Find projects with any keywords: find all
This find all
command allows you to find projects even if you have forgotten the project name. You can simply try to find the project using words that appear in the description of the project. If you remember the name of the client, you can use the client name to find the project too.
Format: find all KEYWORDS [MORE KEYWORDS]
Examples:
-
find all software food
Returns a list of projects which contains either software or food or both as part of the name/description/client.
Below is a screenshot of what a successful find all command will look like.
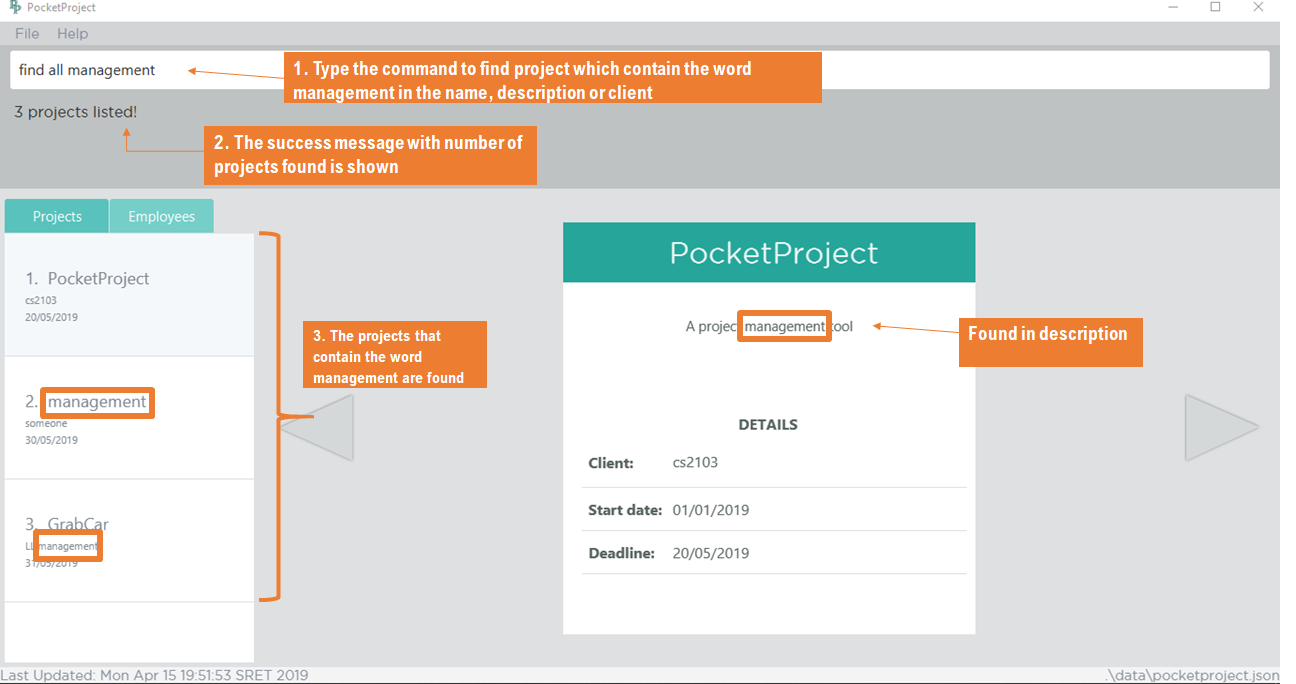
find all management
commandEdits name/client/deadline/description of a project: edit project info
You can use this command to edit the general details of an existing project such as project name, deadline, client and description which are shown on the summary panel of the projects.
This command can be used to change the default description that is assigned to the project when the project is added. |
Format: edit project PROJECT_NAME info [n/name] [c/client] [d/deadline] [desc/description]
Examples:
-
edit project Apollo info n/Gemini c/John d/12/09/2020 desc/An application for project management
Change project Apollo name to Gemini, client to John, deadline to 12/09/2020 and the description to 'An application for project management'
Both the fixed date format and the flexible date can be used here to edit the deadline. |
|
Note that the start date is unmodifiable and the deadline should be after the start date of the project and also after the date of the latest milestone in the project. |
|
Note that 'info' is a reserved word so it is not accepted as part of the argument for the description of the project (e.g. 'm/project info' is not accepted). |
Below is the before and after screenshots of when the command edit project PocketProject info desc/A project management tool d/20/05/2019
is entered.
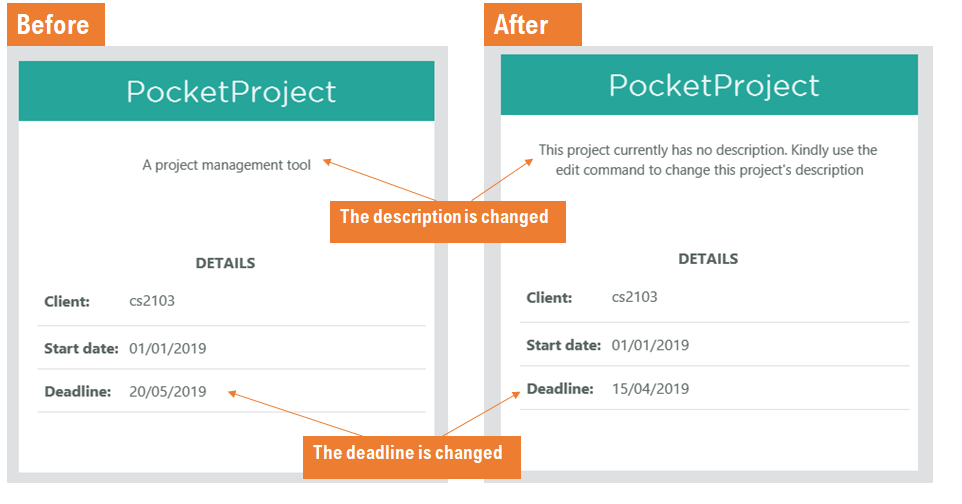
edit project PocketProjcet info desc/A project management tool d/20/05/2019
commandEdit milestone of a project: edit project milestone
You can edit the milestone of the existing project whenever there are changes made to it.
Format: edit project PROJECT_NAME milestone MILESTONE_INDEX [m/milestone] [d/date]
Examples:
-
edit project Apollo milestone 2 m/Implement edit command d/09/04/2019
Change the milestone at index 2 in Apollo to 'Implement edit command (09/04/2019)'
The date of the milestone must be between the start date and the deadline of the project |
|
A blank input for milestone description will not be accepted as an input. (e.g. |
|
Note that 'milestone' is a reserved word so it is not accepted as part of the argument for the description of the milestone (e.g. 'm/A milestone for ui' is not accepted). |
Below is the before and after screenshot of how the command should work. The command
edit project Orbital milestone 1 m/Version 1.1 refactoring d/03/08/2019
will be used to show
this.
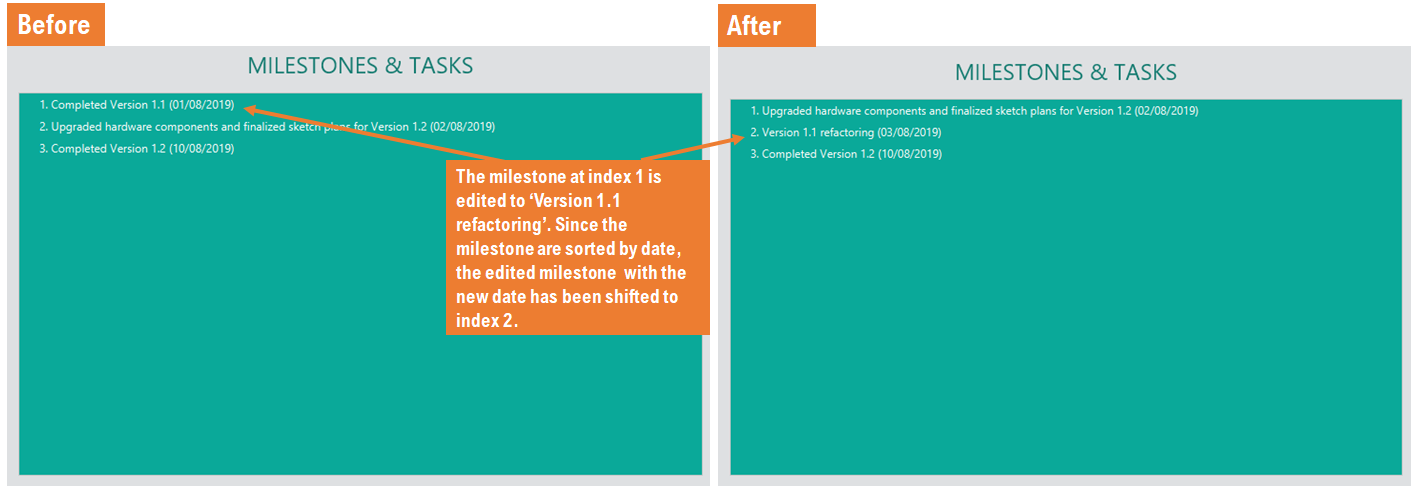
edit project Orbital milestone 1 m/Version 1.1 refactoring d/03/08/2019
Contributions to the Developer Guide
This section includes documentation on implementation of the find
and edit
command that I worked on.
Find Employee/Project/Skill/All feature
Current Implementation
For the current find
feature, there are 5 main commands that the user can execute:
-
find employee [KEYWORDS]
- display employees with name containing keywords -
find project [KEYWORDS]
- display projects with name containing keywords -
find skill [KEYWORDS]
- display employees who has skills matching the keywords -
find all [KEYWORDS]
- display projects which contains words matching the keywords -
find deadline KEYWORD
- display projects which contains deadline before the keyword
The FindCommandParser
differentiates these 5 commands based on the COMMAND_KEYWORDS
entered by the users (e.g. employee/project/skill/all/deadline) as illustrated in the diagram below.
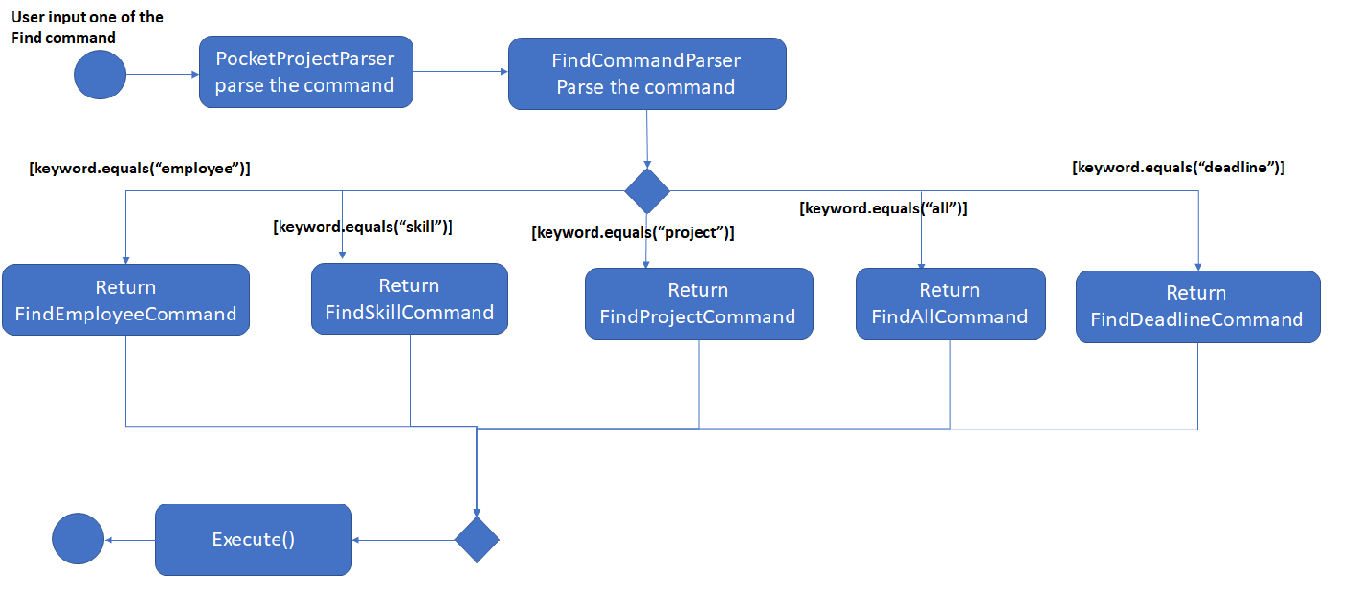
FindCommandParser
The following sequence diagram shows how the find employee
command works:
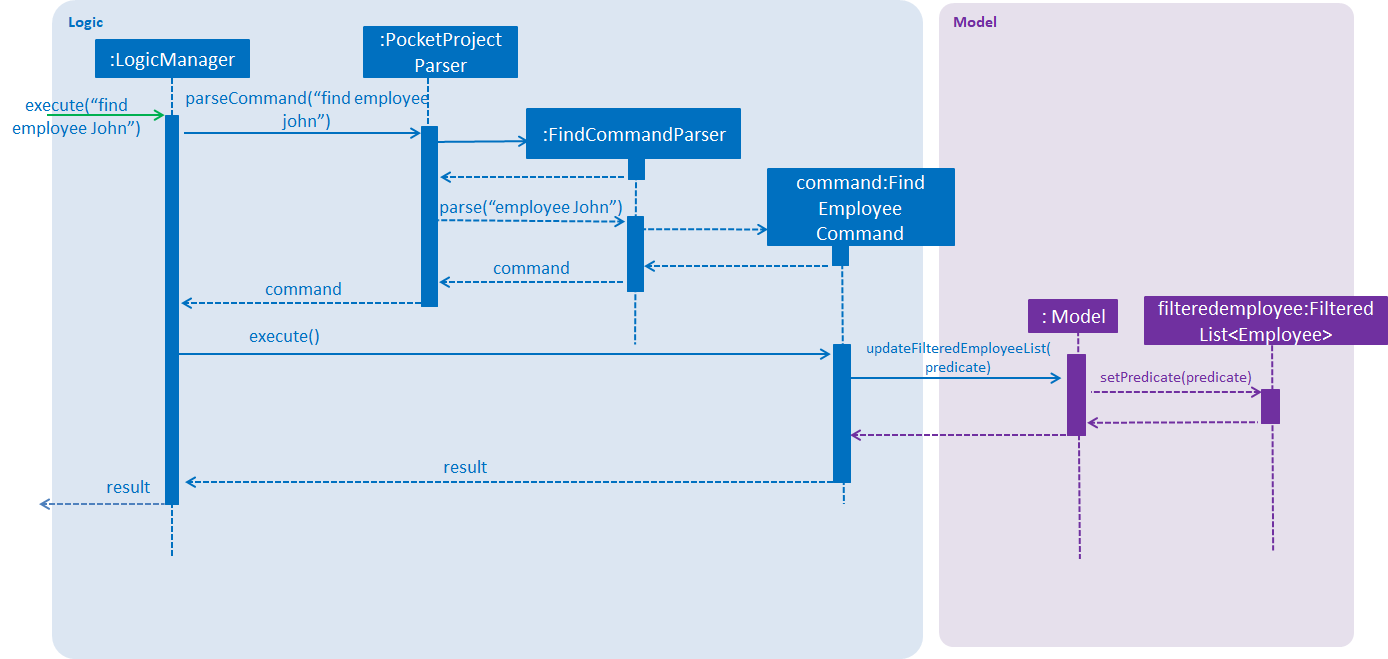
find employee John
commandUsage Scenario example for find employee
:
-
User executes
find employee John
to look for employees whose names contains the word 'John'. -
PocketProjectParser
will parse and identify the command as aFindCommand
and pass on the argumentemployee John
toFindCommandParser
. -
FindCommandParser
identify the command based on theCOMMAND_KEYWORD
'employee'. -
find employee
command is executed. Employees with name consisting the keywords are filtered out from the employeelist. The filtering is done through the use ofProjectNameContainsKeywordPredicate
that checks through the names of the employee int the application. -
Finally, the
filteredEmployees
list which is the list displayed on the ui is updated.
The working mechanism for find project
is similar to find employee
. Instead of updating the filteredEmployees
, filteredProjects
is updated instead to display the list of projects with name consisting the keywords.
For find all
command, the predicate is used to check for the matching keywords in the project name , description and client, thereby filtering out any projects that contains the keyword in these components of the project.
For find skill
command, the List<Skills>skills
from the employee is retrieved as a String
to match against the keywords. Then the matched employees are filtered and displayed on the ui.
find deadline
uses the ProjectContainsDeadlinePredicate
to compare the deadline of the project against the supplied deadline and filter out the projects with deadline earlier or same as the deadline supplied by the user.
Design Considerations
Aspect: How find
command is parsed
Alternative 1: Have a separate FindCommandParser
to parse the command (current implementation)
Alternative 2: Let PocketProjectParser
handle parsing of all the find
commands
Using Alternative 1 is better because it provides better abstraction as the details of the 5 different find commands will be hidden away as just a single FindCommand.
Hence it is more in line with OOP principles. There will also be less clutter in PocketProjectParser
as there are already a lot of other commands being parsed.
Edit Project feature
Current Implementation
This feature allows editing of several components which are under project and is mainly facilitated by the model component. This feature supports 3 main commands:
-
edit project PROJECT_NAME info [n/name] [c/client] [d/deadline] [desc/description]
- allow editing of project’s name, deadline, description -
edit project PROJECT_NAME milestone MILESTONE_INDEX [m/milestone] [d/date]
- allow editing of details of project milestone such as milestone description and date -
edit project PROJECT_NAME userstory USERSTORY_INDEX STORY
- allow editing of details of project’s userstory (explained here [Edit user story command])
The EditProjectCommand Parser
differentiate these 3 commands based on the COMMAND_KEYWORD
which are info, milestone and userstory.
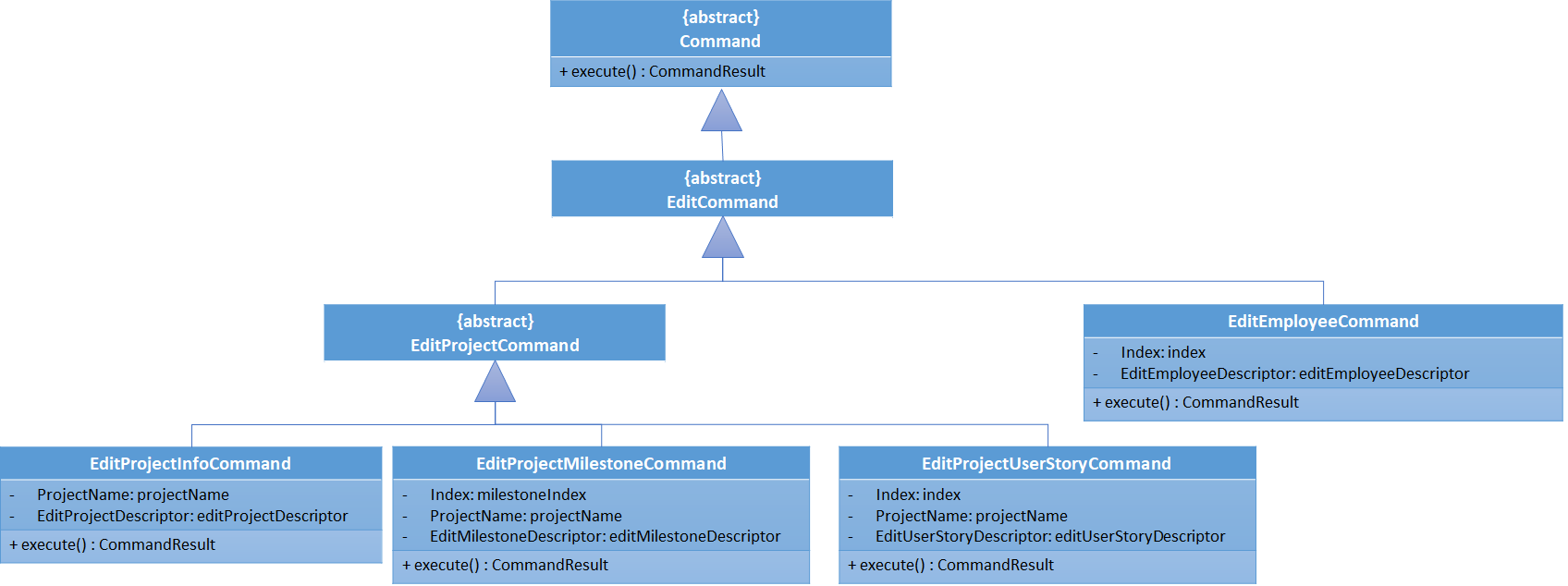
EditCommand
All these 3 commands are inherited from the EditProjectCommand
as shown in the diagram above. This allows flexibility as more edit project command can be easily added when there are new components added to the project.
The following sequence diagram shows how edit project info
command works:
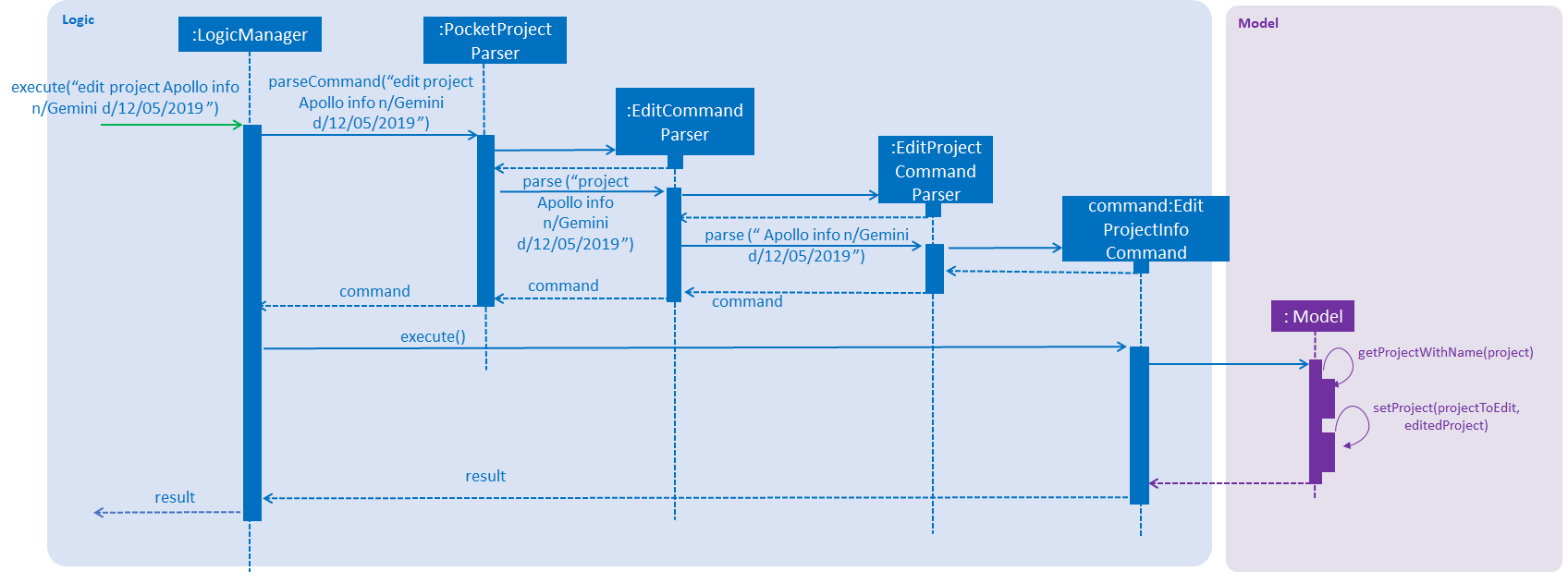
edit project Apollo info n/Gemini d/12/05/2019
Given below is the explanation of what happens when the user enter the edit project Apollo info n/Gemini d/12/05/2019
command based on the diagram above:
-
User executes
edit project Apollo info n/Gemini d/12/05/2019
to edit name and deadline of the project named Apollo. -
PocketProjectParser
will identify the command as anEditCommand
based on the wordedit
and pass on the argument to the EditCommandParser. -
Similarly the arguments will continue to parse through
EditCommandParser
andEditProjectCommandParser
which will identify the command asEditProjectInfoCommand
and return it back to theLogicManager
-
LogicManager
then execute the command. Validity of the arguments are checked here as well. The methodModel#setProject(projectToEdit, editedProject)
is called to replace the old project with the edited project which contains the new parameters. This method also update the list of project names in the employees who are assigned to project Apollo. -
Finally, the project Apollo is edited and the updated details are shown on the UI.
If the arguments provided are invalid, a |
The working mechanism behind EditProjectMilestoneCommand
is similar as well. Instead of replacing the whole project, only the milestone list in the project is edited by replacing the milestone at the given index through the Model#setMilestone(milestoneToEdit, editedMilestone)
method.
Design Consideration
Aspect how to structure the classes under edit command
Alternative 1: Have a single edit project command that allow users to edit all the parts of the project in one command
Alternative 2: Have several separate commands that edit different parts of the project (current implementation)
For alternative 1, the user will not need to remember many commands just to edit the project. However since there are a lot of components in the project, different prefixes will be needed for different components so that the parser can identify which component to edit. There are also overlapping attributes in the components such as milestone date and project deadline or milestone description and project description. Therefore it is going to be messy in terms of code as well as in terms of usage of the command.
Even though alternative 2 involves having to remember different commands, it can be implemented in a way so that the commands are intuitive to the user. For instance,
the command format for current implementation is quite intuitive. The user just need to specify edit project
then add in name of the project to edit and the components the user wish to edit.
Thus, alternative 2 is chosen as it is less confusing both in term of code and usage and can be tweaked to make the command user-friendly.